August 4, 2016
Diamond Pattern
Diamond Pattern
#include<iostream.h>
#include<conio.h>{
clrscr();
int i,j,n,k;
cin>>n; /* Enter Odd No. Only*/
for(i=0;i<n;i+=2)
{
for(j=0;j<n-i;j++)
{
cout<<" ";
}
for(k=1;k<i;k++)
{
cout<<" *";
}
cout<<endl;
}
for(i=0;i<n;i+=2)
{
for(j=1;j<=i;j++)
{
cout<<" ";}
for(k=0;k<n-i;k++)
{
cout<<"* ";
}
cout<<endl;
}
getch();
}
Calculating temperature in Celcius or Fahrenheit
Calculating temperature in Celsius or Fahrenheit
#include<iostream.h>#include<conio.h>
#include<math.h>
void main()
{
clrscr();
double t,ct,choice;
cout<<"Temperature Conversion Menu"<<"\n";
cout<<"1. Fahrenheit to Celsius"<<"\n";
cout<<"2. Celsius to Fahrenheit"<<"\n";
cout<<"Enter choice (1-2):\t";
cin>>choice;
if (choice==1)
{ cout<<"\n"<<"Enter temperature in Fahrenheit:\t";
cin>>t;
ct=(t-32)/1.8;
cout<<"Temperature in Celsius is "<<ct<<"\n";
}
else
{ cout<<"\n"<<"Enter temperature in Celsius:\t";
cin>>t;
ct=(1.8*t)+32;
cout<<"Temperature in Fahrenheit is :\t"<<ct;
}
getch();
}
Example of Switch Statement
Example of Switch Statement
#include<iostream.h>
#include<conio.h>void main()
{
clrscr();
float a,b,r;
char ch;
cout<<"Enter First Number :\t";
cin>>a;
cout<<"Enter Second Number :\t";
cin>>b;
cout<<"Enter operator (+,-,*,/,%) :\t";
cin>>ch;
cout<<"\n";
switch (ch)
{
case '+': r=a+b;
cout<<"\n Adding :\t"<<r;
break;
case '-': r=a-b;
cout<<"\n Subtracting :\t"<<r;
break;
case '*': r=a*b;
cout<<"\n Multipling :\t"<<r;
break;
case '/': if(b==0)
cout<<"\nDivide by ZERO";
else
r=b/a;
cout<<"\n Dividing :\t"<<r;
break;
case '%': if(b==0)
cout<<"\nDivide by ZERO";
else
{
int res,q;
q=b/a;
res=b - (q*a);
res=r;
cout<<"\n Modulus :\t"<<r;
}
break;
default : cout<<"\nCheck your CHOICE";
}
getch();
}
Prime Number
Prime Number
#include<iostream.h>
#include<conio.h>
void main()
{
clrscr();
int flag=1,num,i;
cin>>num;
for(i=2;i<num;i++)
{
if(num%i==0)
{ flag=0;break;}
}
if(flag==1)
cout<<"\nPrime";
else
cout<<"\nNot Prime";
getch();
}
#include<conio.h>
void main()
{
clrscr();
int flag=1,num,i;
cin>>num;
for(i=2;i<num;i++)
{
if(num%i==0)
{ flag=0;break;}
}
if(flag==1)
cout<<"\nPrime";
else
cout<<"\nNot Prime";
getch();
}
FACTORIAL
FACTORIAL
#include<iostream.h>
#include<conio.h>
#include<math.h>
void main()
{
clrscr();
int a,b,c=1,x,z;
cout<<"Enter Number:\t";
cin>>a;
if(a%2==0)
{
cout<<"\nEven";
a=b;
while(b)
{
z*=b;
--b;
}
cout<<"\nThe Factorial of"<<b<<"is"<<z<<"\n";
}else
{
for(x=2;x<z;x++)
{
if(z%x==0)
{ z=0;break;
}
}
if(z==1)
{
cout<<"\nOdd Prime";
}else
{
cout<<"\nNot a Prime but ODD";
}
}
getch();
}
#include<conio.h>
#include<math.h>
void main()
{
clrscr();
int a,b,c=1,x,z;
cout<<"Enter Number:\t";
cin>>a;
if(a%2==0)
{
cout<<"\nEven";
a=b;
while(b)
{
z*=b;
--b;
}
cout<<"\nThe Factorial of"<<b<<"is"<<z<<"\n";
}else
{
for(x=2;x<z;x++)
{
if(z%x==0)
{ z=0;break;
}
}
if(z==1)
{
cout<<"\nOdd Prime";
}else
{
cout<<"\nNot a Prime but ODD";
}
}
getch();
}
STRING PALINDROME
String is Palindrome or not.
#include<iostream.h>#include<conio.h>
#include<stdio.h>
void main()
{
clrscr();
char str[80];
int i=0,flag=0,j,len;
cout<<"Enter String :\t";
gets(str);
while(str[i]!='\0')
{
len++;
i++;
}
for(i=0,j=len-1;i<j;i++,j--)
{
if(str[i]!=str[j])
{
flag=1;break;}
}
if(flag==0)
cout<<"PALINDROME";
else
cout<<"NOT PALINDROME";
getch();
}
August 2, 2016
Comparing String without using the function (strcmp)
Comparing String without using the function (strcmp)
#include<iostream.h>#include<conio.h>
#include<stdio.h>
void main()
{
clrscr();
char a[80],b[80];
int i=0,j=0,flag=0,len,l;
cout<<"Enter first string :\t";
gets(a);
cout<<"Enter second string :\t";
gets(b);
while(a[i]!='\0')
{
len++;
i++;
}
while(b[j]!='\0')
{
l++;
j++;
}
for(i=0,j=0;a[i]!='\0'||b[j]!='\0';i++,j++)
{
if((int)a[i]>(int)b[j])
{flag=1;break;}
else
if((int)a[i]<(int)b[j])
{flag=2;break;}
}
if(flag==1)
cout<<"1";
else
if(flag==2)
cout<<"-1";
else
cout<<"0";
getch();
}
LCM
LCM
#include<iostream.h>#include<conio.h>
void main()
{
clrscr();
int a,b,lcm;
cout<<"Enter two no.'s :\t";
cin>>a>>b;
if (a%b==0)
{
lcm=b;
}
else
if(a%2==0 && b%2==0)
{
lcm=(a*b)/2;
}
else
if(a%2==0||b%2==0)
{
lcm=a*b;
}
else
{lcm=a*b;}
cout<<"LCM = "<<lcm;
getch();
}
Example Of IF Statement
Example Of IF Statement
#include<iostream.h>
#include<conio.h>
void main()
{
clrscr();
int age;
cout<<"Enter Age:\t";
cin>>age;
if(age>18);
{
cout<<"Yes";
cout<<"\nEligible for Voting";
}else
{
cout<<"\nSorry";
cout<<"\nNot eligible";
}
getch();
}
Calculating FORCE
C++ Program for Calculating FORCE
#include<iostream.h>
#include<conio.h>
void main()
{
clrscr();
float d,t,v,m,a,f;
cout<<"\nEnter Distance:\t";
cin>>d;
cout<<"\nEnter Time:\t";
cin>>t;
v=d/t;
cout<<"Velocity:\t"<<v;
a=v/t;
cout<<"\nAccleration:\t"<<a;
cout<<"\nEnter Mass:\t";
cin>>m;
f=m*a;
cout<<"\nForce:\t"<<f;
getch();
}
SWAPPING
C++ PROGRAM FOR SWAPPING
#include<iostream.h>
#include<conio.h>
void main()
{
clrscr();
int x,y,z;
cout<<"x:\t";
cin>>x;
cout<<"\ny:\t";
cin>>y;
z=x-y;
cout<<"\nz:\t"<<z;
x=x-z;
cout<<"\nAfter swaping x=\t"<<x;
y=y+z;
cout<<"\nAfter swaping y=\t"<<y;
getch();
}
#include<conio.h>
void main()
{
clrscr();
int x,y,z;
cout<<"x:\t";
cin>>x;
cout<<"\ny:\t";
cin>>y;
z=x-y;
cout<<"\nz:\t"<<z;
x=x-z;
cout<<"\nAfter swaping x=\t"<<x;
y=y+z;
cout<<"\nAfter swaping y=\t"<<y;
getch();
}
August 1, 2016
Curved Surface Area of Cone
#include<iostream.h>
#include<conio.h>
void main()
{
clrscr();
int l,r,csa;
cout<<"Enter Radius:\t";
cin>>r;
cout<<"\nEnter Slant Height:\t";
cin>>l;
csa=3.14*r*l;
cout<<"\nCurved Surface Area:\t"<<csa;
getch();
}
#include<conio.h>
void main()
{
clrscr();
int l,r,csa;
cout<<"Enter Radius:\t";
cin>>r;
cout<<"\nEnter Slant Height:\t";
cin>>l;
csa=3.14*r*l;
cout<<"\nCurved Surface Area:\t"<<csa;
getch();
}
July 31, 2016
July 27, 2016
How to increase your instgram follower without any download
Hi everyone, Myself anubhav today i am going to tell you about a greate trick to get increasement in your instam follower. Today's time everyone wants to get popularity or maintain a class betwen his/her circle incuding friends and relatives. So they try to get it but directly it is not soo easy it may time taking. For this there are too many applications or hacks available but most kf them are spamming and even harmful for your cell. So the question what should our next step, today i will tell you a trick. May be many of you don't believe it firstly but i recommend you to use it only time follow all step as tells in post i am sure definitely you see a lot more changes in your follower speed and it requires noo downloads so there is not any risk. So now come to know about the trick
Get follower without any download
- Open your Instagram account
- Search for geteasily
- Now follow it and also folllow them who are followed by that i mean follow open that following option and follow all of them
- Wait for 5 min you will see there are some people started following you
- Now 15 after unfollow them and follow again and again as much as you want
Believe it i use it and get about 100 follower just in 2 hours
Share your experience here...
July 25, 2016
Download Official Prisma Free
Hi guys welcome to techtro we are here to give you another trick which make a class of you between your relatives . After seeing all your loves we are sharing another great tricks that works for you and definitely follow this. We are going to know that how to download or install prisma app for android which is previously used only for IOS. Before this you have to know about prisma
Share 😄
Is any problem behind all these just comment us we will be happy to help you
keep visiting and don't forget to bookmarks us.
What is PRISMA
Prisma is a photo editing app created by a Russian programmar Alexey Moiseenkov . Apart from cool filter it is minimilastic, clean and easy to use. This app leads you artistic effect shows creativity in art. At this time it has about 33 filters to use.
How to use PRISMA
- Download and install prisma there is no attachment with social world it don't ask you for your email or phone number
- just open there will be option for take a photo direct or get that from sd card or internal
- After it choose a photo and wait to load it sfter it done. Save it! and share with your loved ones...
Many peoples try to install it but problem is how to get it ?, where you can find this app so the answer is very simple just open given link and get that
it is available at Playstore ❤️
https://play.google.com/store/apps/details?id=com.neuralprismaShare 😄
Is any problem behind all these just comment us we will be happy to help you
keep visiting and don't forget to bookmarks us.
July 24, 2016
Techtro Free Recharge Giveway ( SHARE & WIN 100 RECHARGE)
Hey guys, i hope you all are enjoying our deals, offers & recharge tricks . If you have missed those deals dont worry , we are starting our 1st giveway contest . You can earn 100 rs recharge paytm code in just 3 easy steps. Checkout more details about this giveway below :
HOW TO PARTICIPATE IN THIS GIVEWAY
- Just click here & like our facebook page .
- Share our fb page post on your timeline ( Privacy must be public)
- After sharing on FB take a screenshot & Whatsapp us on +917784871648 (Whatsapp broadcast channel)
- Recharge codes will be provided within few hours once our team verifies.
SO FRIENDS HURRY UP & GRAB THIS EXCITING GIVEWAY & STAY TUNED WITH US FOR MORE OFFERS & DEALS IN FUTURE !!!
July 22, 2016
Atletico de Kolkata to build Rs.500 crore football academy! It will consist of a Football Stadium and 120-room hotel.
RP-Sanjiv Goenka Group to float Rs 500 crore football academy
Declining to detail more, Goenka said the "self-sustaining" project will be on less than 50 acres of land
The RP-Sanjiv Goenka Group, which co-owns Indian Super League (ISL) franchise Atletico de Kolkata, is coming up with a Rs 500 crore football academy in the city's vicinity, a top company official said on Friday.
Group chairman Sanjiv Goenka said the proposal for the project, comprising a football academy and a stadium along with a 120-room hotel, has been submitted to the West Bengal government for its approval.
"The land has been identified. It will be a football academy with a small stadium to start with, which will be developed later. There will also be a 120-room hotel and training facility. The total investment is Rs. 500 crore," Goenka told mediapersons in Kolkata.
"We have submitted the proposal to the state government. As soon as we get the approval we will move ahead with the project. The first half of the project (stadium and academy) will be completed within one year from the date of approval," he said.
Declining to detail more, Goenka said the "self-sustaining" project will be on less than 50 acres of land and will come up in the city's vicinity.
"The self-sustaining venture will be the first of its kind in the country. We have got all the plans ready. International firms were hired for preparing the project details," said Goenka adding that the investment for the project will come from the company's CSR stream and funding from family members.
Goenka, who also co-owns the Indian Premier League (IPL) franchise Rising Pune Supergiants, said the company was exploring avenues to expand its sporting ventures with the ISL franchise to become profitable in 2018.
"Our franchise in the IPL is only till next year, It will not even break even next year but the performance will be way better. As regards football, we are expected to become profitable year after next year," added Goenka.
Mcent dominos offer (Unlimited trick)
Steps to Follow:
Firstly download mCent App
Install and Open the app
Now in the Offer section, you can see download Domino’s App and place an order to Get Rs.36
Now Download Domino’s App and Place your Order
If you have Domino’s pizza vochers, you can place order
Don’t worry, if you have not Domino’s vocher, you can place fake order with any amount
Done. That’s it!! You will get Rs.36 mCent in just 5 minutes
Unlimited Trick:
Firstly, Clear data of mCent App
Now change the android I’d, Imei and Google advertising I’d
Now Login to your another mCent account and follow the above steps
Do this steps again and again to earn mCent cash
Join our WhatsApp broadcast now and get exclusive updates on scripts and tricks.Simply send ADD to “7784871648” and you will be added within few hours.
INDIAN Teenager Trip to Spain For LA LIGA
Good News For Indian Football fans
Bengaluru Boy Ishan Pandita got a offer from newly promoted La Liga club Club Deportivo Leganés & set to sign a deal with them in coming days.
The Talented striker Ishan Pandita who went to Spain last year & playing for Under-18 team of UD Almeria till now.
Read The interview Of Ishan Pandita
How did you come to Almeria all the way from India?
Ishan Pandita: When I was 16, I moved to Madrid for a year and played there. A friend of mine then helped me come in contact with Almeria and after a couple of talks, I arrived in Almeria in November 2015.
Where do you see yourself 3 years from now?
Ishan: Playing in a top division team – Spanish or English.
How popular is football in India?
Ishan: It’s growing a lot with ISL (Indian Super League). We have a good level now and a lot of money. The attendance(per game) gets up to 50, 60 thousand people and matches are also broadcasted on television.
What ‘s the biggest difference between football in India and in Spain?
Ishan: There’s not much of a difference anymore. Kalu Uche, who is now playingfor Almeria in Segunda Division, used toplay in ISL so I don’t think the levels are that far off.
Was it hard to adapt to Spanish football when you arrived to Spain?
Ishan: When I first came to Madrid it was obviously difficult. The technical level in Spain is very high. Coming to Almeria was a bit easier after spending a year in Madrid.
What’s the most important quality for a striker in you opinion?
Ishan: Technical abilities, strength and knowing when to move – basically assessing the whole game. It’s important to use your brain and play smart. Being calm and composed in front of goal is important too.
What’s your advice to players outside of Europe? Should they join academies and pay to train with the best?
Ishan: I think it’s better to pay to train in Spain than being in India, because the level of playing is much higher in Spain.
Who’s your favourite player?
Ishan: I like Luis Suarez. Also Neymar, Sturridge and Coutinho.
If Deal Will Done then ishan pandita will first indian To play in La Liga
Bengaluru Boy Ishan Pandita got a offer from newly promoted La Liga club Club Deportivo Leganés & set to sign a deal with them in coming days.
The Talented striker Ishan Pandita who went to Spain last year & playing for Under-18 team of UD Almeria till now.
Read The interview Of Ishan Pandita
How did you come to Almeria all the way from India?
Ishan Pandita: When I was 16, I moved to Madrid for a year and played there. A friend of mine then helped me come in contact with Almeria and after a couple of talks, I arrived in Almeria in November 2015.
Where do you see yourself 3 years from now?
Ishan: Playing in a top division team – Spanish or English.
How popular is football in India?
Ishan: It’s growing a lot with ISL (Indian Super League). We have a good level now and a lot of money. The attendance(per game) gets up to 50, 60 thousand people and matches are also broadcasted on television.
What ‘s the biggest difference between football in India and in Spain?
Ishan: There’s not much of a difference anymore. Kalu Uche, who is now playingfor Almeria in Segunda Division, used toplay in ISL so I don’t think the levels are that far off.
Was it hard to adapt to Spanish football when you arrived to Spain?
Ishan: When I first came to Madrid it was obviously difficult. The technical level in Spain is very high. Coming to Almeria was a bit easier after spending a year in Madrid.
What’s the most important quality for a striker in you opinion?
Ishan: Technical abilities, strength and knowing when to move – basically assessing the whole game. It’s important to use your brain and play smart. Being calm and composed in front of goal is important too.
What’s your advice to players outside of Europe? Should they join academies and pay to train with the best?
Ishan: I think it’s better to pay to train in Spain than being in India, because the level of playing is much higher in Spain.
Who’s your favourite player?
Ishan: I like Luis Suarez. Also Neymar, Sturridge and Coutinho.
If Deal Will Done then ishan pandita will first indian To play in La Liga

July 21, 2016
Learn C++ With #Chitra_karakoti
HI GUYZ we have decided to start C++ tutorials in our site for student of class 11 & 12 cbse specially and for other people also , we will counter all the major parts of C++ from noob to pro
we will also provide you program code the author of this post will be #chitra_karakoti
you all can see the posts of C++ in the C++ arena link is provided in menu which is provided to you just below our logo the menu is not good but we are working on it .
thanks guyz for reading this its just a notification post to you all we will start this on coming sunday
any question ping me on +917784871648 or comment down
for more tips tricks send "sub" to +917784871648
we will also provide you program code the author of this post will be #chitra_karakoti
you all can see the posts of C++ in the C++ arena link is provided in menu which is provided to you just below our logo the menu is not good but we are working on it .
thanks guyz for reading this its just a notification post to you all we will start this on coming sunday
any question ping me on +917784871648 or comment down
for more tips tricks send "sub" to +917784871648
July 20, 2016
How to get instant like on your facebook page
Facebook is a utility where we share our thoughts and daily activities with our friends or relatives. Now Facebook page which started for for business becomes as a craze between teens to show how they popular in social world. All of them tries hard to get likes but without a planning they can't able to do that.
Make a event of that (fake or real) to win prises just like given page and invite that to 50 or as your wish you want
Why people want likes
To show your are better than next is the property of peraon. Some of them use it to get advertise his/her business. Many of them use paid method but some are still search for free way to get likes
They googled it but there is a lackness of getting the accurate results but today this poat helps you alot
How to get likes
Get likes on a fb page for free is laborious and time spending but results definitely show
- Create a page of you if you not still have no one. (If you have an old offline page i reccomend for a new one)
- Invite your all friend which added in your friendlist and try to convince them to like your page.
- Share it in different social networks like twitter, google+ etc.
- Now the main things to post that viral pics that person like to share.
- Get your page id n. (ex- 415931835190801) 15 digit code
How to get page code : just open your page and copy the adress of that page it seems like ( https://www, facebook, com/php?pageid=415931835190801)
just copy that digit
- Stay online i suggest you to give all your friends feedback as fast as can
- Always try to post between 1pm to 3pm for more likes.
- its not important to post more but important to post quality or shareable post.
- Use that code as @[415931835190801:] comment for like, the above digit will turns as the name of that page
Make a event of that (fake or real) to win prises just like given page and invite that to 50 or as your wish you want
Facebook always share that which are post by more places. Keeping all these facts in your mind you can get likes on your pages
Don't forget to give your special reaction. If there any problem in understanding just comment ans for more bookmarks us and keep visiting.
How to get Reliance JIO 4G Sim without having LYF Handset
Reliance and Samsung have launched Jio 4G Preview SIM offer.
This Reliance Jio 4G Preview Offer will be available for selected Samsung smartphones(Described Below) and will let you enjoy unlimited Free Internet With Super Fast 4G, Free SMS, Free Calling , Free Video streaming and EveryThing Free and Jio Premium apps for 90 days/3 months.
Handsets which it Supports
For getting 4G Sim first you have to buy 4G Handset or if you having then see your phone model number in our list and then follow all given steps.
Handset or Mobile Phones List
- Samsung Galaxy Note Edge
- Samsung Galaxy S6 Edge Plus
- Samsung Galaxy Note Edge
- Samsung Galaxy Core Prime 4G
- Samsung Galaxy Note 4
- Samsung Galaxy Note 5
- Samsung Galaxy A5
- Samsung Galaxy A7
- Samsung Galaxy A8
- Samsung Galaxy S6
- Samsung Galaxy A7 (A710FD)
- Samsung S7 Edge
- Samsung A8 VE
- Samsung Galaxy J5
- Samsung Galaxy J2
- Samsung Galaxy J7
- Samsung Galaxy On5
- Samsung Samsung On7
- Sony Xperia Z5 Dual
- Sony Xperia Z5 Premium Dual
- LG Spirit 4G
- LG Google Nexus 5x
- LG G4 Stylus 4G
- LG G3 4G LTE 32GB
- ZTE Blade S6
- ZTE Blade S6 Plus
- Lenovo Vibe Shot
- Lenovo A6000 Plus
- Intex Aqua Ace Mini
- Intex Aqua 4G Strong
- Intex Aqua 4G
- Karbonn A71
- Karbonn Aura
- Motorola Moto G (3rd Gen)
- Motorola Moto E 2nd Gen
- Motorola Moto E 2nd Gen
- Motorola G Turbo
- Micromax Yu Yunique
- Micromax Yu Yuphoria
- Micromax Canvas Sliver 5 (Q450)
- Micromax Canvas Amaze (Q491)
- Xiaomi Mi5
- Xiaomi Redmi Note 3 (H3A)
- Alcatel Pop3
- OPPO F1
- Black BerryPriv (STV100-3)
- Infocus M370
- Infocus M370I
- GIONEEE life S6
- Lenovo Vibe Shot
Trick to Get Reliance JIO 4G Sim
- Download My Jio App from playstore.
- After Downloading My Jio App just open it & check that JIO 4G is available in your location or not.
- If yes, then you have to Generate the offer code,which includes bar code. (Samsung-Reliance-Jio-Preview-offer)
- Go to Nearby Reliance Digital Store and collect your Free JIO 4G SIM at a Reliance Digital or Dx Mini Store after submitting the identity, bar code, address proof and your photograph.
- That’s it. They will give you Reliance Jio 4G Sim.
How to Activate Reliance JIO 4G Sim
- First of all insert JIO 4G Sim into above mention phone
- After inserting just dial 1977 to activate your sim
- Now Customer Care will pick up the phone and ask you to provide details of the owner of the sim
- Then just give details.
- Finally you will getting message after activation of your sim and you will also get email also.
So Just enjoy & stay tuned for more updates !
July 18, 2016
Youtube Unlimited Subscribers FREE FREE FREE
Hi guyz my name is neeraj kholiya
here we are with the new trick or you can say new way to get youtube subscribers . there is a app name #live_sub_count which gives you free subscribers its actually work on the principle of S2S follow the steps given below
Requirements -
- An android Mobile
- Internet connection
- Brain ;-)

Step 1 - Download the app name #Live_sub_count { pic given above}
Step 2 - Login with your id
Step 3- Earn coins By subscribing other channel
Note - Subscribing 1 channel will give you 4 $ coins
every campaign start after 1 hour
Step 4 - You can use earned coins to get subscriber 1 sub = 12 $coins
Bang use after every 1 hour to get unlimited subscribers
If your are riche rich can spend real money so below is the rate chart

There are others offers also to get coins
Important Note you can use more than 1 account
you can also share earn coins to your friends or you can get of your friends use this unlimited trick and get millions of subscribers
your question - y u had not got
ans - got about it today
we believe in sharing is caring
share us TECHTRO
for more tips and tricks send "sub" to +917784871648 ( whatsapp )
July 10, 2016
July 9, 2016
Pokemon Go guide - Catching, Battling And Everything You Need To Know
hi guyz i am neeraj kholiya here we are to share some tips and tricks of #Pokemon_go
it is now available for iOS and Android and trainers young {xda ot ;-) } and old {others} can go out into the world and catch your favorite Pokémon.
But before you go out you’ll want to brush up some of the basics and features of the new mobile game so you can become a Pokémon Master that much quicker. So let’s start off with the basics.
BASICS
When you boot up Pokémon Go for the first time, you’ll have to customize your trainer, name him/her and then choose your starter Pokémon. You choose from either Bulbasaur, Charmander or Squirtle, the classic Gen 1 starters.
Once you’ve caught your first Pokémon the real adventure begins with the interface showing your trainer walking on a Google Maps-esque layout. The GPS on your smartphone will pinpoint your location.
On the map you can see landmarks that represent PokeStops and Gyms. You won’t be able to access them until you get closer to their destination, or in the case of Gyms at a certain level.
You’ll also see rustling grass. If you walk towards it a Pokémon will appear and you will attempt to catch it.
If you look on the bottom right corner of your screen, you can pull up the menu that tells you what Pokemon are near you.
Each Pokemon will have zero to three footprints underneath them. Zero means they are really close and three means they are the furthest away.
CATCHING POKEMON
Catching a Pokémon in Pokémon Go is very different from the main games. You don’t use your party Pokémon to weaken a wild one, instead your catch rate is determined by a few factors.
The Pokémon’s CP level, the type of Poké Ball you used, your throwing technique, and other factors come into play when determining whether the Pokémon can be successfully caught.
Other Pokémon will simply run away so be sure to be quick.
Your throwing technique is probably the one thing you’ll want to master. Your angle and force behind your throwing (which is a simple flick on the touchscreen) is a big factor. And, of course, you’ll want to hit the Pokémon with the Poke Ball.
If you have the AR turned on, you'll want to center the Pokemon on the screen. Keep your phone steady as you throw but if you want an easier experience, turn the AR off and the Pokemon will remain centered.
You'll also notice a ring inside a circle where the Pokemon is. When that ring (normally green) is at its smallest is when you'll want to throw your Poke Ball.
Various habitats will determine what Pokémon is found. For example, water Pokémon will be found near bodies of water and beaches. Use the Nearby feature on the bottom of the screen to see which Pokémon are around.
The item Incense will draw Pokémon toward your for 30 minutes, so be sure to use them when you are having trouble finding Pokémon in the wild.
POKEMON TRAITS
Now that you’ve caught a Pokémon, you can take a look at what it can do. A Pokémon’s Command Points (CP) determines how well they fight in battle. The higher the CP the better they fare.
You’ll also notice that some Pokémon of the same species will have varying CP when they are captured. Keep that in mind when you are training them up as you’ll want to keep the highest CP Pokémon.
The higher your Trainer’s level, the higher CP Pokémon will appear.
LEVELING UP/EVOLUTION
If you want to level up your Pokémon, then you’ll have to use the Stardust gained from capturing Pokémon to do so.
Evolving Pokémon takes an extra step that involves the Transfer function, the Professor, along with Pokémon-specific candies.
We go more in-depth on leveling and evolving on our guide, here.
POKESTOPS
As i mentioned PokeStops before and they are different areas around you that will give items and even Eggs.
The markers on the map will show blue squares, once you are close enough you just spin the medal and items will drop. There is a cooldown for each PokeStop so be sure to check back to certain landmarks to see when you can get more items from the same area.
EGGS
When gathering items at PokéStops, you may find Eggs that will eventually hatch into Pokémon. To hatch these Eggs, you'll need to walk around a certain distance.
The farther you have to walk, the rarer the Pokémon you'll find inside when it finally hatches.
You’ll need Incubators to hatch Eggs. There is one provided for you so only one Egg can be hatched at a time unless you purchase another one.
ITEMS
Like the Incubator and the Incense, there are plenty of different items in Pokémon Go. PokeStops are a great way to get more Poke Balls and other items. Serebii has a great listing of the differing items you can find, so check that out.
But like many mobile games, there are microtransactions that players can choose to purchase with real money. You can purchase Poke Dollars to get more items.
You’ll gain Poke Dollars by leveling up and performing other tasks in-game but if you want to shorten the process than you can purchase using money. Check out the chart for microtransactions below.
BATTLING/GYMS
After your trainer reaches Level 5 you can join a team (either Red, Blue or Yellow) and start to battle Gyms.
Once you join a team, you can assign Pokémon you've caught to an open Gym or to a Gym where a team member has placed one of his or her Pokémon. Like PokéStops, Gyms can be found at real locations in the world.
Only one Pokémon per player will be set at a particular Gym.
If you want to tackle another team’s Gym, you’ll want to check out its stats. Prestige determines how difficult a Gym will be to take it over. Training Pokémon at your Gym will increase its Prestige, while losing battles to other teams' Pokémon will lower it.
Training your Pokémon is simple, just visit a Gym that's already controlled by your team. Once there, you can train by battling against your own team's Pokémon. Defeat all of them and your Gym's Prestige will go up, making it more difficult to be defeated by another team.
If the Gym's Prestige is reduced to zero, the defending team loses control of the Gym, and you or another player can then take control of it by assigning a Pokémon to protect it.
Gyms are a collaborative effort in Pokémon Go so be sure to check back to your team’s Gyms once in awhile and be sure to train your own Pokémon.
If you want more in-depth details about Gyms and how they work, come over to our Gym Guide.
if i many any important thing of game comment down
for more tips tricks movies games send "sub" to 7784871648
How To Mirror Your Android Screen To PC - Wifi, USB | No Root
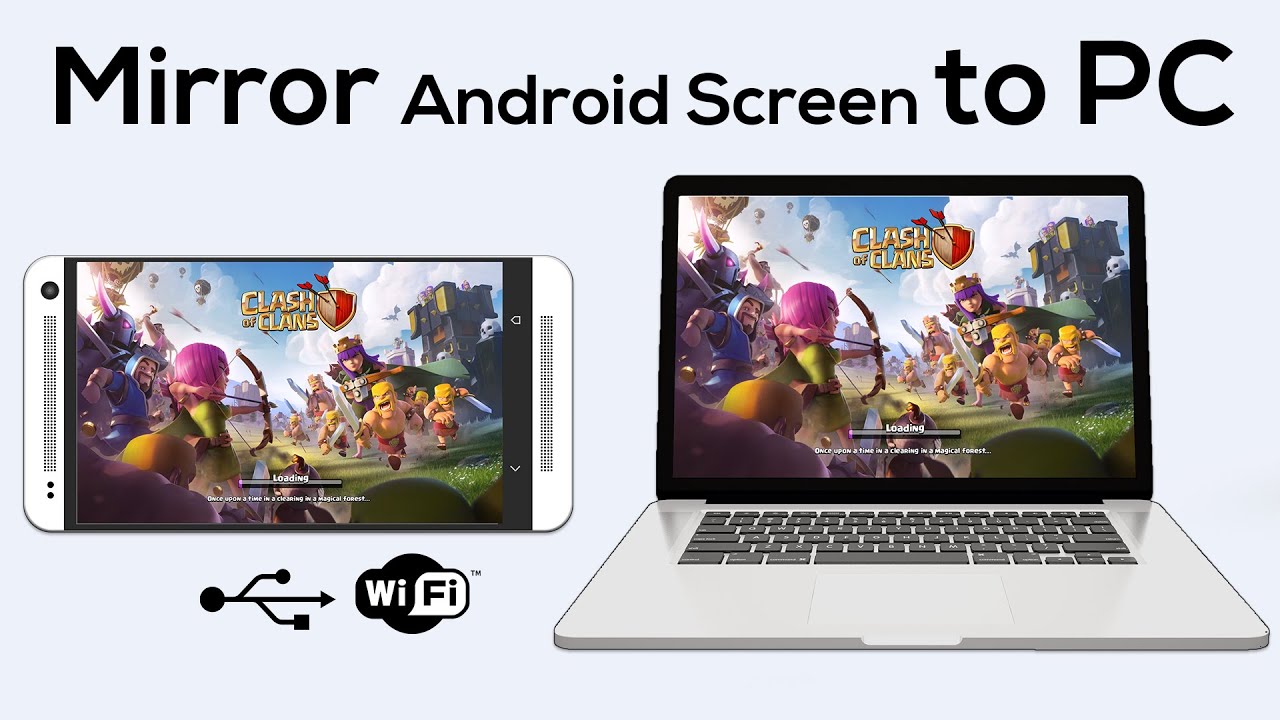
Do you want to Mirror your Android Device screen to your PC over wifi or USB? If yes then stay tuned to this article to find How.
Sometimes there are situations, where you want to use your Phone content on your PC or TV, In this situation we usually transfer the files to PC and then use it. Now with MirrorGo software you can easily Mirror your Android Device screen to your PC.
We are using wifi and USB as a medium to do this, and also, this does not require any root access.
Before going in, we need a software for doing this.You can download it from the Link given below.
Now let's Start.
Some Features of MirrorGo:
Download Link:.
You can download MirrorGo software from their official website.Link: Official website
How To Mirror?
Step 1: Download MirrorGo software from the Link given above.
Step 2: Install it.
Step 3: Once Installed, Open it.
Step 4: Now adjust the screen Resolution of your choice or leave setting default.
Step 5: Now connect your Android Phone to your PC using USB Cable or wifi.
(If you are connecting via wifi then, Make sure that both the PC and Phone is connected to same wifi network.)
(If you are connecting via USB Cable, then make sure that USB Debugging is enabled on your Phone.)
Step 6: Wait for the setup.
Step 7: Once done, the Mirroring process will start
Now whatever things you do in your Phone gets reflected exactly in your PC, You may Play a game, watch a Video, or even chat with your Friend.
Check: [Guide] How to Install Phoenix OS on your PC/Laptop? (UEFI / Legacy)
- We also have some additional options like Recording screen, Take a snapshot etc.
Hope you guys find this article useful, Comment below your feedbacks and Doubts.

Subscribe to:
Posts (Atom)